Use your own Cart methods
Cart flow can be sometimes pretty complicated. Caast provide an easy way to mimic your add to cart functionnality without the need of custom development but sometimes, we simply cannot execute this action.
Hopefully, integrate your own flow while keeping the full Caast experience is an easy task, this tutorial is here to help you throught this journey.
Enable cart method to only send event
First of all, you will need to ping our team in order to disable the default add to cart methods inside Caast. Nevertheless, if you have control over the Caast configuration, simply set send_only_events
to true (cf screen below)
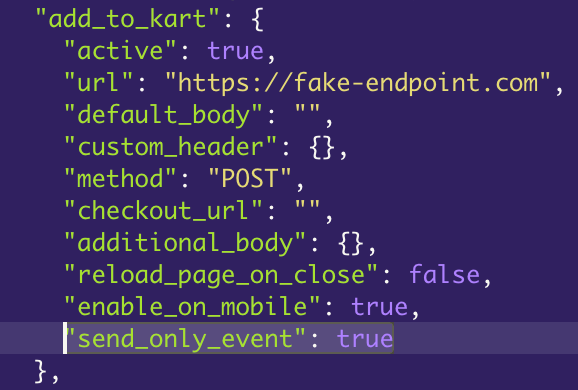
Catch the add to cart event
Now Caast will let you do the dirty work in order to add an item to your cart. In order to do so, you will have to start listening to a specific event, the onBasketAdd event.
var checkCaastExist = setInterval(() => {
if (typeof window.caast !== 'undefined') {
if (typeof window.caastIsListeningCart === 'undefined') {
caast
.on('onBasketAdd', (data) => {
console.log('Yeepikai ! Received data =>', { data });
})
.then(() => {
window.caastIsListeningCart = true;
})
.catch((error) => console.error(error));
}
}
}, 100);
Implement you own add to cart method
Hurray ! You are now subscribed to our add to cart event, which means that you can now implement your own method.
For the sake of simplicity, we will suppose you have an available method named add_to_cart
, yes really innovative function name, and this method simply needs a SKU in order to add to your cart.
We expose and explain the available object information regarding the product because you may find this data interesting
var checkCaastExist = setInterval(() => {
if (typeof window.caast !== 'undefined') {
if (typeof window.caastIsListeningCart === 'undefined') {
caast
.on('onBasketAdd', (data) => {
/**
* This is the Product Reference we have in our database, it is supposed to match the ones in your own database
**/
const SKU = data.product_ref;
/**
* This is an array of the selected product variation. A variation can be a color, a size or any other selectable attribute
**/
const VARIATION_SELECTED = data.variation_selected;
/**
* This is all the data we have about the added product
**/
const PRODUCT_DETAIL = data.product_detailed;
/**
* This is a merged attributes version of the added product and the data coming from the variation
**/
const PRODUCT_DETAIL_WITH_VARIATION = data.current_product_variation;
/*
* Call your own method, we are in a listener,
* do not hesitate to check if your method is available in this context, better safe than sorry
*
**/
if (typeof add_to_cart !== 'undefined') {
add_to_cart(SKU);
}
})
.then(() => {
window.caastIsListeningCart = true;
})
.catch((error) => console.error(error));
}
}
}, 100);
Boom ! You now have full control of the add to cart method.
We are using the PiP and I don't see my cart being updated
Glad to ear that you like the Picture In Picture feature, it's a really nice one. First of all, take a deep breath, your code is perfect, so are you, but we need to implement additionnal code in our listener.
Why ? Because the PiP is building an iframe of the current page over everything, and the cart you are updating is in fact, hidden by this iframe.
How ? We will need to tell the iframe to refresh the cart because the parent thread have updated it. Once again for the sake of simplicity, we will suppose you have an available method named refresh_cart
, yes again a really innovative function name.
Let's do this:
var checkCaastExist = setInterval(() => {
if (typeof window.caast !== 'undefined') {
if (typeof window.caastIsListeningCart === 'undefined') {
/**
* This code will run in both main and iframe page, so we first need to identify
* in which context we are running
*
* Hopefully, we also expose an easy way to do this
**/
const caastIsInIframe = caast.isInIframe();
/**
* We will need to communicate between the main thread and the iframe, the BroadcastChannel
* feature is a great way to do this easily
*
* https://developer.mozilla.org/en-US/docs/Web/API/Broadcast_Channel_API
*
**/
const broadEvent = new BroadcastChannel('caast_cart_channel');
/**
* The broadcast event name, you can customize it
**/
const broadEventName = 'caast::update_cart';
/**
* This condition will only run inside the iframe
**/
if (caastIsInIframe) {
/**
* Start listening to the broadCastChannel
**/
broadEvent.onmessage = (event) => {
/**
* We receive information about iframe parent adding to cart
*/
if (event.data === broadEventName) {
/*
* Call your refresh_cart method, we are in a listener,
* do not hesitate to check if your method is available in this context, better safe than sorry
*
**/
if (typeof refresh_cart === 'function') {
refresh_cart();
}
}
};
window.caastIsListeningCart = true;
/**
* This condition will only run on the main Caast thread, outside the iframe
**/
} else {
caast
.on('onBasketAdd', (data) => {
/**
* This is the Product Reference we have in our database, it is supposed to match the ones in your own database
**/
const SKU = data.product_ref;
/**
* This is an array of the selected product variation. A variation can be a color, a size or any other selectable attribute
**/
const VARIATION_SELECTED = data.variation_selected;
/**
* This is all the data we have about the added product
**/
const PRODUCT_DETAIL = data.product_detailed;
/**
* This is a merged attributes version of the added product and the data coming from the variation
**/
const PRODUCT_DETAIL_WITH_VARIATION = data.current_product_variation;
/*
* Call your own method, we are in a listener,
* do not hesitate to check if your method is available in this context, better safe than sorry
*
**/
if (typeof add_to_cart !== 'undefined') {
add_to_cart(SKU);
/**
* Broadcast 'caast::update_cart' to the channel in order to ping that it has been updated
**/
broadEvent.postMessage(broadEventName);
}
})
.then(() => {
window.caastIsListeningCart = true;
})
.catch((error) => console.error(error));
}
}
}
}, 100);
And voilà ! You now have a fully working custom add to cart solution. If these tutorial do not cover your usecase, please contact our technical team and we will help you solve the problem you are encountering.